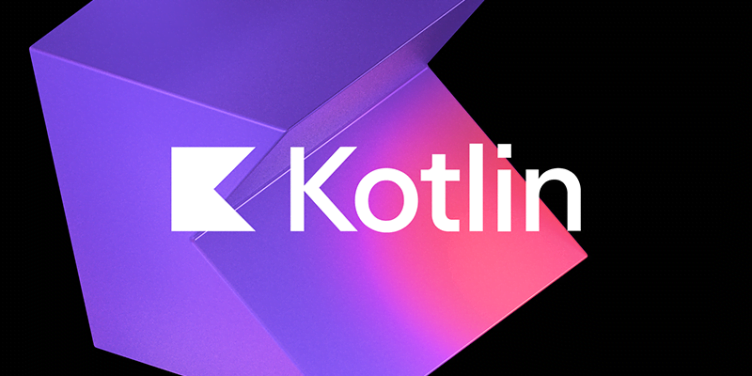
Kotlin has become increasingly popular over the past few years. It’s an open-source, statically-typed language compatible with Java and known for being concise, expressive, and safe. In this article, I’ll dive into why Kotlin is an excellent choice for programming and why you should consider using it for your next project.
If you want to learn more about programming and technology, I invite you to connect with me on LinkedIn and check out my other articles and library, KTweet. I’m available on Discord.
1. Kotlin’s simplicity
One of the most significant advantages of Kotlin is its simplicity. If you’re from a Java background, you’ll find the syntax is familiar and easy to understand. However, even if you’re new to programming, Kotlin is easy to pick up, and you’ll find that you can start building functional applications in no time.
Variable Declaration
val name: String = "John"
var age: Int = 30
The above code declares a variable name
of type String
and initializes it with the value "John"
. It also declares a variable age
of type Int
and initializes it with the value 30
. The 'val'
keyword is used for immutable variables, whereas the 'var'
keyword is used for mutable variables.
Function Declaration
fun add(x: Int, y: Int): Int {
return x + y
}
The above code declares a function add
that takes two parameters x
and y
of type Int
and returns their sum as an Int
. The function body is enclosed in curly braces, and the ‘return'
keyword is used to return a value.
Kotlin’s simplicity makes it easy for developers to focus on solving problems rather than worrying about language complexities. Its concise syntax and expressiveness help reduce the boilerplate code needed to accomplish tasks, resulting in more readable and maintainable code.
Additionally, Kotlin provides a wealth of resources and tools for learning, such as the official Kotlin documentation, tutorials, and IDE support. These resources make it easy for developers to start and continue learning and improving their skills. With Kotlin, you can quickly and easily build applications for various platforms, including Android, desktop, and web applications, making it a universal language for developers of all levels.
2. Kotlin’s interoperability with Java
One of the most significant benefits of Kotlin is that it’s interoperable with Java. This means you can use Kotlin code in Java projects and vice versa. This makes integrating Kotlin into existing codebases and working with Java libraries easy.
Java Code
public class MyClass {
public void sayHello() {
System.out.println("Hello, World!");
}
}
Kotlin Code
fun main() {
val obj = MyClass()
obj.sayHello()
}
The above code demonstrates how Kotlin can use Java classes seamlessly. We have a simple Java class MyClass
that has a method “sayHello()
“, which prints “Hello, World!” to the console. In the Kotlin code, we create an instance of MyClass
and call the sayHello()
method on it. The output will be “Hello, World!” printed to the console.
Kotlin also allows you to use Java libraries in your Kotlin projects without any additional setup. You can import the Java library classes in your Kotlin code and use them as you would in a Java project.
Another advantage of Kotlin’s interoperability with Java is that it makes it easy to migrate from Java to Kotlin. If you have an existing Java codebase, you can gradually start converting your code to Kotlin while still using the current Java code. This makes the migration process less daunting and more manageable, as you can start using Kotlin for new features while maintaining the existing Java codebase.
Overall, Kotlin’s interoperability with Java makes it a versatile language that can be used in various projects, from small to large-scale applications. It allows developers to leverage the strengths of both languages and provides a smooth transition path for those looking to migrate from Java to Kotlin.
3. Kotlin’s conciseness and expressiveness
Kotlin is designed to be straightforward and has many features that make it easier to write code quickly. For example, it has a type inference system that automatically infers the type of variables, so you don’t have to declare them explicitly. It also has a functional programming style, making writing expressive and concise code easier.
val name = "John"
val age = 30
The above code declares two variables name
and age
. However, we did not have to specify these variables’ data types explicitly. Kotlin’s type inference system automatically infers the type based on the value assigned to the variable. In this case, name
is inferred to be of type String
, and age
is inferred to be of type Int
.
val numbers = listOf(1, 2, 3, 4, 5)
val evenNumbers = numbers.filter { it % 2 == 0 }
The above code uses a lambda expression to filter even numbers from a list of numbers. The filter
function is called on the numbers
list, and the lambda expression { it % 2 == 0 }
is used to filter only even numbers. The resulting evenNumbers
list will contain [2, 4]
.
Kotlin’s concise syntax and expressiveness make writing code easy to read and maintain. Its functional programming style allows developers to write more declarative and less verbose code, reducing the boilerplate code needed to accomplish tasks. This makes it easier to write complex applications with fewer lines of code, resulting in code that is more concise and easier to understand.
4. Kotlin’s safety features
Kotlin has many features that make it safe and less prone to errors. For example, it has a null safety system that eliminates the possibility of null pointer exceptions. It also has an extensive type system that catches errors at compile-time, so you can catch errors before running your code.
Null Safety
var name: String? = null
val length = name?.length // safe call operator
In the above code, we have declared a nullable String
variable name
. This means that name
can either contain a String
value or be null. We then use the safe call operator ?.
to call the length
property on name
. If name
is null, the safe call operator will return null instead of throwing a null pointer exception.
Type System
fun divide(a: Int, b: Int): Int {
require(b != 0) { "Division by zero is not allowed" }
return a / b
}
In the above code, we have a function divide
that takes two integers a
and b
as input and returns their quotient. However, before performing the division, we use the require
function to ensure that b
is not zero. If b
is zero, the require
function will throw an exception with the message “Division by zero is not allowed.” This ensures that we catch errors at compile-time and prevent the possibility of runtime errors.
Kotlin’s safety features make it less prone to errors and provide developers with tools to catch errors early in development. This results in more reliable, maintainable code and easier to debug. Additionally, Kotlin’s safety features help developers avoid common pitfalls such as null pointer exceptions, which frustrate many developers.
Overall, Kotlin’s safety features make it a language that prioritizes developers’ well-being and their code’s reliability. Its safety features help developers write code that is less prone to errors and easier to maintain, resulting in more reliable and robust applications.
5. Kotlin’s tooling and community support
Kotlin has great tooling, including an excellent IDE called IntelliJ IDEA, which provides code completion, debugging, and other valuable features. It also has a growing community of developers who contribute to open-source projects, answer questions on forums like Stack Overflow, and organize Kotlin-specific events.
Tooling:
- IntelliJ IDEA: JetBrains, the company behind Kotlin, also developed IntelliJ IDEA, which is widely considered one of the best IDEs for development. It provides excellent code completion, debugging, and refactoring tools, making it easier for developers to write, test, and debug their code.
- Android Studio: Kotlin is also the official language for Android app development. This makes it easy to create Android apps with Kotlin and take advantage of its features.
- Gradle: Gradle is the build system of choice for many Kotlin projects and has excellent support. It provides plugins for compiling, testing, and packaging code, making it easier for developers to build and distribute their applications.
Community Support:
- Open-Source Projects: Kotlin has many open-source projects, ranging from libraries and frameworks to tools and utilities. Many of these projects are hosted on GitHub and have active communities of contributors and users.
- Events: There are also many Kotlin-specific events, such as KotlinConf and Kotlin Nights, which provide opportunities for developers to learn about Kotlin, meet other developers, and share their experiences.
Kotlin’s tooling and community support make it an attractive language for developers who value productivity, reliability, and community. Its tooling helps developers write, test, and debug their code more efficiently, while its community support provides opportunities to learn from others and contribute to open-source projects. Overall, Kotlin’s tooling and community support contribute to a vibrant and growing ecosystem that makes Kotlin an excellent choice for modern software development.
Kotlin’s Advantages
One of the most significant advantages of Kotlin is that it’s constantly evolving, with regular updates and improvements. JetBrains, the company behind Kotlin, is committed to making Kotlin the best language it can be. They work closely with the Kotlin community to continuously improve the language and its ecosystem. This means that Kotlin constantly improves with new features, bug fixes, and optimizations, making it even more productive and enjoyable.
Overall, Kotlin is a powerful and practical language that offers many benefits for developers. Its ease of use, interoperability, conciseness, expressiveness, safety and excellent tooling, and community support make it an attractive choice for many projects. Whether a beginner or an experienced developer, Kotlin is a language worth exploring and considering for your next project.